A Beginner’s Guide to Frontend Development
In today’s digital age, creating a compelling and intuitive user experience is essential for the success of any web application. At the heart of crafting these experiences are the core technologies of frontend development: HTML, CSS, and JavaScript. These technologies form the backbone of web design and interactivity, enabling developers to create visually appealing and dynamic websites. Let’s explore the basics of these technologies and their real-world applications and build a simple website using our newly learned skills.
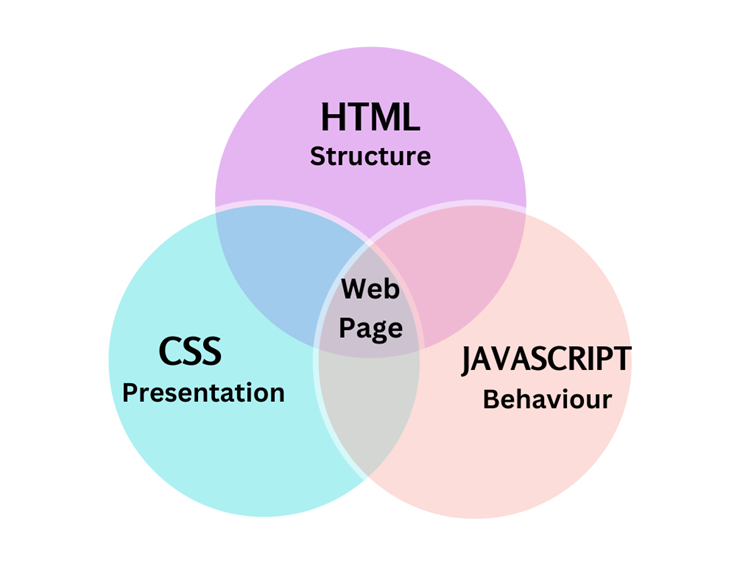
HTML: Structuring the Web
HTML (HyperText Markup Language) is the foundation of web development, providing the structure and content of a webpage. Think of HTML as the skeleton of a website, where each element is a building block that defines the layout and organization of content.
Key Features:
- Elements and Tags: HTML uses elements encapsulated in tags to create the structure, such as <header>, <nav>, <section>, <article>, and <footer>.
- Attributes: Tags can have attributes that provide additional information, such as id, class etc.
- Hyperlinks: The <a> tag is used to create links to other webpages or resources.
An HTML document has two main tags: <head> and <body>. The <head> element serves as a container for metadata and is placed between the opening <html> tag and the <body> tag. Metadata is data about the HTML document. Metadata is not displayed. The <body> element contains all the contents of an HTML document, such as headings, paragraphs, images, hyperlinks, tables, lists, etc.
Here’s a simple HTML snippet that structures a basic webpage with a header, navigation, content section, and footer:
index.html
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
<section id="home">
<h2>Home Section</h2>
<p>This is the home section of the webpage.</p>
</section>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
In this example, the <header> tag is used to define the header section of the webpage, containing a welcome message. The <nav> tag defines a navigation menu with links. The <section> tag represents the main content area, and the <footer> tag defines the footer with copyright information. The <a> tag is also used to provide hyperlinks of the home, about and contact pages. They can be clicked to navigate to the respective pages when made.
In practice, HTML is essential for defining the basic structure of a webpage, ensuring that content is organized logically and accessible to users and search engines alike.
CSS: Styling the Web
CSS (Cascading Style Sheets) is used to style and layout web pages. It allows developers to create visually appealing sites by applying styles to HTML elements. CSS controls the presentation layer, enhancing the user experience through design and layout.
Key Features:
- Selectors: Select HTML elements to style, such as body, .class-name, and #id.
- Properties and Values: Define styles using properties like color, font-size, margin, and padding.
- Responsive Design: Media queries enable responsive design, making websites adaptable to different screen sizes.
To implement CSS, we create another file named style.css where we define all our styles and then link it to our HTML document using <link> tag by providing the path to the style.css file. Since style.css is in the same folder, we simply provide the name of the file.
Here’s an example of CSS that styles the HTML structure we defined earlier:
style.css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
text-align: center;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
}
nav{
margin: auto;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 10px;
}
a{
text-decoration: none;
color: black;
}
section {
padding: 20px;
background-color: white;
margin: 20px;
border-radius: 5px;
}
footer {
text-align: center;
padding: 1em 0;
background-color: #4CAF50;
color: white;
position: fixed;
width: 100%;
bottom: 0;
}
button{
height: 40px;
width: 100px;
border-radius: 12px;
background-color: #4CAF50;
This CSS styles the body of the webpage to have a specific font and background color. The header is styled with a background color, text color, and padding. The navigation menu is styled to remove bullet points and display links inline. The sections are styled with padding, background color, margin, and border-radius to create a card-like appearance. The footer is styled to be fixed at the bottom of the page.
To apply the newly created stylesheet, add the following <link> tag within the <head> section of your index.html file:
<link rel = “stylesheet” href = “style.css”>
index.html
<head>
<title>My First Webpage</title>
<link rel="stylesheet" href="style.css" />
</head>
This line connects your HTML document to the style definitions stored in the style.css file.
CSS is crucial for transforming a plain HTML document into a polished, visually appealing webpage. It ensures that websites look good on all devices, from desktops to smartphones.
JavaScript: Bringing Interactivity
JavaScript is the programming language that enables dynamic behavior and interactivity on websites. It can manipulate HTML and CSS, handle events, validate forms, and much more.
Key Features:
- DOM Manipulation: JavaScript can interact with and modify the Document Object Model (DOM) to dynamically update content.
- Event Handling: Respond to user actions like clicks, mouse movements, and keyboard inputs.
- APIs: Integrate with various web APIs to extend functionality, such as fetching data from a server.
JavaScript adds life to a webpage, allowing developers to create rich, interactive experiences. It is the key to building features like image sliders, form validations, and real-time content updates.
Here’s a simple JavaScript example that displays an alert when a button is clicked:
script.js
// JavaScript to display an alert when a button is clicked
document.addEventListener('DOMContentLoaded', function() {
document.getElementById('myButton').addEventListener('click', function() {
alert('Button was clicked!');
});
});
This code sets up a button that, when clicked, triggers a JavaScript function to display an alert message. The addEventListener method is used to listen for the click event on the button with the ID myButton.
Incorporate the following HTML code to include the JavaScript file and add a button to test the JavaScript functionality:
index.html
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
<link rel="stylesheet" href="style.css" />
<script src="script.js" defer></script>
</head>
<body>
<!-- Other content here -->
<button id="myButton">Click Me!</button>
<footer>
<!-- Footer content here -->
</footer>
</body>
</html>
The complete code
Here’s the complete code for your implementation:
index.html
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
<link rel="stylesheet" href="style.css" />
<script src="script.js" defer></script>
</head>
<body>
<header>
<h2>Welcome to My Website</h2>
</header>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
<section id="home">
<h2>Home Section</h2>
<p>This is the home section of the webpage.</p>
</section>
<button id="myButton">Click Me!</button>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
style.css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
text-align: center;
}
header {
background-color: #4CAF50;
color: white;
padding: 1em 0;
}
nav{
margin: auto;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 10px;
}
a{
text-decoration: none;
color: black;
}
section {
padding: 20px;
background-color: white;
margin: 20px;
border-radius: 5px;
}
footer {
text-align: center;
padding: 1em 0;
background-color: #4CAF50;
color: white;
position: fixed;
width: 100%;
bottom: 0;
}
button{
height: 40px;
width: 100px;
border-radius: 12px;
background-color: #4CAF50;
}
script.js
document.addEventListener('DOMContentLoaded', function() {
document.getElementById('myButton').addEventListener('click', function() {
alert('Button was clicked!');
});
});
Final output:
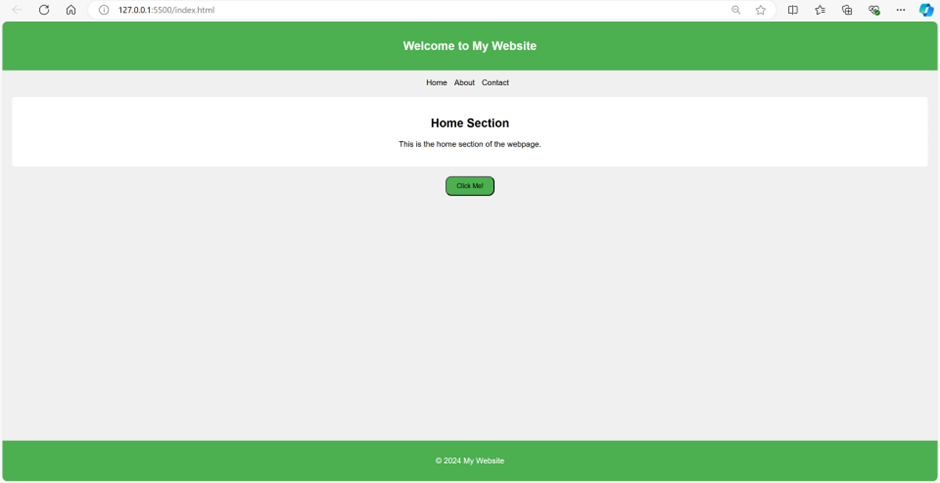
Real-World Applications
HTML, CSS, and JavaScript are used in countless real-world applications, from simple personal blogs to complex web applications. Here are some examples of how these technologies are used:
- Personal Websites and Blogs: HTML structures the content, CSS styles it, and JavaScript adds interactivity like comments and social media sharing.
- E-commerce Sites: HTML creates the product pages, CSS designs the layout, and JavaScript handles the shopping cart and checkout process.
- Web Applications: Platforms like Google Maps or Twitter use HTML for structure, CSS for styling, and JavaScript for dynamic content updates and user interactions.
Conclusion
Frontend development with HTML, CSS, and JavaScript is essential for creating engaging and user-friendly web applications. By mastering these core technologies, developers can build sophisticated and dynamic websites that provide a seamless user experience. Whether you’re creating a simple blog or a complex web application like a chatbot, HTML, CSS, and JavaScript are the fundamental tools you’ll use to bring your vision to life.
By: Aditya Singh